Nibbles
In this part of the Jython Swing programming tutorial, we will create a Nibbles game clone.Nibbles is an older classic video game. It was first created in late 70s. Later it was brought to PCs. In this game the player controls a snake. The objective is to eat as many apples as possible. Each time the snake eats an apple, its body grows. The snake must avoid the walls and its own body.
Development
The size of each of the joints of a snake is 10px. The snake is controlled with the cursor keys. Initially, the snake has three joints. The game starts immediately. When the game is finished, we display "Game Over" message in the center of the window.import randomFirst we will define some constants used in our game.
from java.awt import Color
from java.awt import Font
from java.awt import Toolkit
from java.awt.event import ActionListener
from java.awt.event import KeyEvent
from java.awt.event import KeyListener
from javax.swing import ImageIcon
from javax.swing import JPanel
from javax.swing import Timer
WIDTH = 300
HEIGHT = 300
DOT_SIZE = 10
ALL_DOTS = WIDTH * HEIGHT / (DOT_SIZE * DOT_SIZE)
RAND_POS = 29
DELAY = 140
x = [0] * ALL_DOTS
y = [0] * ALL_DOTS
class Board(JPanel, KeyListener, ActionListener):
def __init__(self):
super(Board, self).__init__()
self.initUI()
def initUI(self):
self.setBackground(Color.black)
iid = ImageIcon("dot.png")
self.ball = iid.getImage()
iia = ImageIcon("apple.png")
self.apple = iia.getImage()
iih = ImageIcon("head.png")
self.head = iih.getImage()
self.setFocusable(True)
self.addKeyListener(self)
self.initGame()
def initGame(self):
self.left = False
self.right = True
self.up = False
self.down = False
self.inGame = True
self.dots = 3
for i in range(self.dots):
x[i] = 50 - i * 10
y[i] = 50
self.locateApple()
self.timer = Timer(DELAY, self)
self.timer.start()
def paint(self, g):
# due to bug, cannot call super()
JPanel.paint(self, g)
if self.inGame:
self.drawObjects(g)
else:
self.gameOver(g)
def drawObjects(self, g):
g.drawImage(self.apple, self.apple_x, self.apple_y, self)
for z in range(self.dots):
if (z == 0):
g.drawImage(self.head, x[z], y[z], self)
else:
g.drawImage(self.ball, x[z], y[z], self)
Toolkit.getDefaultToolkit().sync()
g.dispose()
def gameOver(self, g):
msg = "Game Over"
small = Font("Helvetica", Font.BOLD, 14)
metr = self.getFontMetrics(small)
g.setColor(Color.white)
g.setFont(small)
g.drawString(msg, (WIDTH - metr.stringWidth(msg)) / 2,
HEIGHT / 2)
def checkApple(self):
if x[0] == self.apple_x and y[0] == self.apple_y:
self.dots = self.dots + 1
self.locateApple()
def move(self):
z = self.dots
while z > 0:
x[z] = x[(z - 1)]
y[z] = y[(z - 1)]
z = z - 1
if self.left:
x[0] -= DOT_SIZE
if self.right:
x[0] += DOT_SIZE
if self.up:
y[0] -= DOT_SIZE
if self.down:
y[0] += DOT_SIZE
def checkCollision(self):
z = self.dots
while z > 0:
if z > 4 and x[0] == x[z] and y[0] == y[z]:
self.inGame = False
z = z - 1
if y[0] > HEIGHT - DOT_SIZE:
self.inGame = False
if y[0] < 0:
self.inGame = False
if x[0] > WIDTH - DOT_SIZE:
self.inGame = False
if x[0] < 0:
self.inGame = False
def locateApple(self):
r = random.randint(0, RAND_POS)
self.apple_x = r * DOT_SIZE
r = random.randint(0, RAND_POS)
self.apple_y = r * DOT_SIZE
# public void actionPerformed(ActionEvent e) {
def actionPerformed(self, e):
if self.inGame:
self.checkApple()
self.checkCollision()
self.move()
else:
self.timer.stop()
self.repaint()
def keyPressed(self, e):
key = e.getKeyCode()
if key == KeyEvent.VK_LEFT and not self.right:
self.left = True
self.up = False
self.down = False
if key == KeyEvent.VK_RIGHT and not self.left:
self.right = True
self.up = False
self.down = False
if key == KeyEvent.VK_UP and not self.down:
self.up = True
self.right = False
self.left = False
if key == KeyEvent.VK_DOWN and not self.up:
self.down = True
self.right = False
self.left = False
The
WIDTH
and HEIGHT
constants determine the size of the Board. The DOT_SIZE
is the size of the apple and the dot of the snake. The ALL_DOTS
constant defines the maximum number of possible dots on the Board. The RAND_POS
constant is used to calculate a random position of an apple. The DELAY
constant determines the speed of the game. x = [0] * ALL_DOTSThese two arrays store x, y coordinates of all possible joints of a snake.
y = [0] * ALL_DOTS
The
initGame()
method initializes variables, loads images and starts a timeout function. def paint(self, g):Inside the
JPanel.paint(self, g)
if self.inGame:
self.drawObjects(g)
else:
self.gameOver(g)
paint()
method, we check the inGame variable. If it is true, we draw our objects. The apple and the snake joints. Otherwise we display "Game over" text. def drawObjects(self, g):The
g.drawImage(self.apple, self.apple_x, self.apple_y, self)
for z in range(self.dots):
if (z == 0):
g.drawImage(self.head, x[z], y[z], self)
else:
g.drawImage(self.ball, x[z], y[z], self)
Toolkit.getDefaultToolkit().sync()
g.dispose()
drawObjects()
method draws the apple and the joints of the snake. The first joint of a snake is its head, which is represented by a red circle. The Toolkit.getDefaultToolkit().sync()
method ensures that the display is up-to-date. It is useful for animation. def checkApple(self):The
if x[0] == self.apple_x and y[0] == self.apple_y:
self.dots = self.dots + 1
self.locateApple()
checkApple()
method checks, if the snake has hit the apple object. If so, we add another snake joint and call the locateApple()
method, which randomly places a new apple object. In the
move()
method we have the key algorithm of the game. To understand it, look at how the snake is moving. You control the head of the snake. You can change its direction with the cursor keys. The rest of the joints move one position up the chain. The second joint moves where the first was, the third joint where the second was etc. while z > 0:This code moves the joints up the chain.
x[z] = x[(z - 1)]
y[z] = y[(z - 1)]
z = z - 1
if self.left:Move the head to the left.
x[0] -= DOT_SIZE
In the
checkCollision()
method, we determine if the snake has hit itself or one of the walls. while z > 0:Finish the game, if the snake hits one of its joints with the head.
if z > 4 and x[0] == x[z] and y[0] == y[z]:
self.inGame = False
z = z - 1
if y[0] > HEIGHT - DOT_SIZE:Finish the game, if the snake hits the bottom of the Board.
self.inGame = False
The
locateApple()
method locates an apple randomly on the board. r = random.randint(0, RAND_POS)We get a random number from 0 to RAND_POS - 1.
self.apple_x = r * DOT_SIZEThese lines set the x, y coordinates of the apple object.
...
self.apple_y = r * DOT_SIZE
def actionPerformed(self, e):Every DELAY ms, the
if self.inGame:
self.checkApple()
self.checkCollision()
self.move()
else:
self.timer.stop()
self.repaint()
actionPerformed()
method is called. If we are in the game, we call three methods, that build the logic of the game. Otherwise we stop the timer. In the
keyPressed()
method of the Board class, we determine the keys that were pressed. if key == KeyEvent.VK_LEFT and not self.right:If we hit the left cursor key, we set
self.left = True
self.up = False
self.down = False
left
variable to true. This variable is used in the move()
method to change coordinates of the snake object. Notice also, that when the snake is heading to the right, we cannot turn immediately to the left. #!/usr/local/bin/jythonIn this class, we set up the Nibbles game.
# -*- coding: utf-8 -*-
"""
ZetCode Jython Swing tutorial
This is a simple Nibbles game
clone.
author: Jan Bodnar
website: zetcode.com
last edited: December 2010
"""
from java.awt import Dimension
from javax.swing import JFrame
from Board import Board
class Nibbles(JFrame):
def __init__(self):
super(Nibbles, self).__init__()
self.initUI()
def initUI(self):
self.board = Board()
self.board.setPreferredSize(Dimension(300, 300))
self.add(self.board)
self.setTitle("Nibbles")
self.pack()
self.setResizable(False)
self.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE)
self.setLocationRelativeTo(None)
self.setVisible(True)
if __name__ == '__main__':
Nibbles()
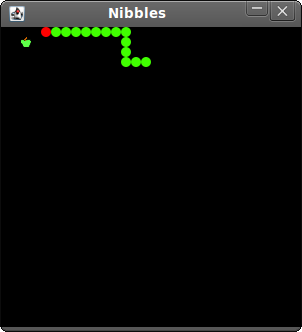
Figure: Nibbles
This was the Nibbles computer game programmed with the Swing library and the Jython programming language.
0 comments:
Post a Comment