Menus And toolbars
In this part of the Java SWT tutorial, we will work with menus & toolbars.A menubar is one of the most common parts of the GUI application. It is a group of commands located in various menus. While in console applications you have to remember all those arcane commands, here we have most of the commands grouped into logical parts. These are accepted standards that further reduce the amount of time spending to learn a new application.
Simple menu
In our first example, we will create a menubar with one file menu. The menu will have only one menu item. By selecting the item the application quits.package com.zetcode;This is a small example with minimal menubar functionality.
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Menu;
import org.eclipse.swt.widgets.MenuItem;
import org.eclipse.swt.widgets.Shell;
/**
* ZetCode Java SWT tutorial
*
* This program creates a simple menu
*
* @author jan bodnar
* website zetcode.com
* last modified June 2009
*/
public class SWTApp {
private Shell shell;
public SWTApp(Display display) {
shell = new Shell(display);
shell.setText("Simple menu");
initUI();
shell.setSize(250, 200);
shell.setLocation(300, 300);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
}
public void initUI() {
Menu menuBar = new Menu(shell, SWT.BAR);
MenuItem cascadeFileMenu = new MenuItem(menuBar, SWT.CASCADE);
cascadeFileMenu.setText("&File");
Menu fileMenu = new Menu(shell, SWT.DROP_DOWN);
cascadeFileMenu.setMenu(fileMenu);
MenuItem exitItem = new MenuItem(fileMenu, SWT.PUSH);
exitItem.setText("&Exit");
shell.setMenuBar(menuBar);
exitItem.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
shell.getDisplay().dispose();
System.exit(0);
}
});
}
public static void main(String[] args) {
Display display = new Display();
new SWTApp(display);
display.dispose();
}
}
Menu menuBar = new Menu(shell, SWT.BAR);Here we create a menu bar.
MenuItem cascadeFileMenu = new MenuItem(menuBar, SWT.CASCADE);Toplevel menu items are cascade menu items.
cascadeFileMenu.setText("&File");
Menu fileMenu = new Menu(shell, SWT.DROP_DOWN);We create a drop down menu.
cascadeFileMenu.setMenu(fileMenu);
MenuItem exitItem = new MenuItem(fileMenu, SWT.PUSH);A push menu item is plugged into a drop down menu.
exitItem.setText("&Exit");
shell.setMenuBar(menuBar);We set a menu bar for our shell.
exitItem.addSelectionListener(new SelectionAdapter() {When we select the exit push menu item, we terminate the application.
@Override
public void widgetSelected(SelectionEvent e) {
shell.getDisplay().dispose();
System.exit(0);
}
});
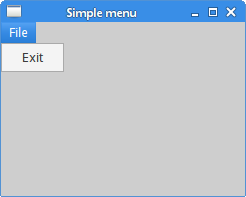
Figure: Simple menu
Submenu
The next example demonstrates how to create a submenu in Java SWT.package com.zetcode;Submenu creation.
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Menu;
import org.eclipse.swt.widgets.MenuItem;
import org.eclipse.swt.widgets.Shell;
/**
* ZetCode Java SWT tutorial
*
* This program creates a submenu
*
* @author jan bodnar
* website zetcode.com
* last modified June 2009
*/
public class SWTApp {
private Shell shell;
public SWTApp(Display display) {
shell = new Shell(display);
shell.setText("Submenu");
initUI();
shell.setSize(250, 200);
shell.setLocation(300, 300);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
}
public void initUI() {
Menu menuBar = new Menu(shell, SWT.BAR);
MenuItem cascadeFileMenu = new MenuItem(menuBar, SWT.CASCADE);
cascadeFileMenu.setText("&File");
Menu fileMenu = new Menu(shell, SWT.DROP_DOWN);
cascadeFileMenu.setMenu(fileMenu);
MenuItem cascadeMenu = new MenuItem(menuBar, SWT.CASCADE);
cascadeMenu.setText("&File");
MenuItem subMenuItem = new MenuItem(fileMenu, SWT.CASCADE);
subMenuItem.setText("Import");
Menu submenu = new Menu(shell, SWT.DROP_DOWN);
subMenuItem.setMenu(submenu);
MenuItem feedItem = new MenuItem(submenu, SWT.PUSH);
feedItem.setText("&Import news feed...");
MenuItem bmarks = new MenuItem(submenu, SWT.PUSH);
bmarks.setText("&Import bookmarks...");
MenuItem mailItem = new MenuItem(submenu, SWT.PUSH);
mailItem.setText("&Import mail...");
MenuItem exitItem = new MenuItem(fileMenu, SWT.PUSH);
exitItem.setText("&Exit");
shell.setMenuBar(menuBar);
exitItem.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
shell.getDisplay().dispose();
System.exit(0);
}
});
}
public static void main(String[] args) {
Display display = new Display();
new SWTApp(display);
display.dispose();
}
}
MenuItem subMenuItem = new MenuItem(fileMenu, SWT.CASCADE);A submenu creation is similar to creating a normal menu. First, we create a cascade menu item. The only difference is the parent widget. This time the parent is the menu object, to which the submenu belongs.
subMenuItem.setText("Import");
MenuItem feedItem = new MenuItem(submenu, SWT.PUSH);We create a push menu item. The parent widget is the submenu object.
feedItem.setText("&Import news feed...");
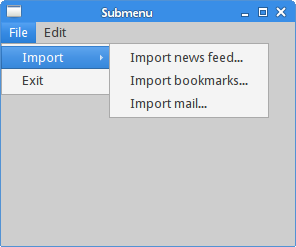
Figure: Submenu
CheckMenuItem
ACheckMenuItem
is a menu item with a check box. It can be used to work with boolean properties. package com.zetcode;In our code example we show a check menu item. If the check box is activated, the statusbar widget is shown. If not, the statusbar is hidden.
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.layout.FormAttachment;
import org.eclipse.swt.layout.FormData;
import org.eclipse.swt.layout.FormLayout;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Event;
import org.eclipse.swt.widgets.Label;
import org.eclipse.swt.widgets.Listener;
import org.eclipse.swt.widgets.Menu;
import org.eclipse.swt.widgets.MenuItem;
import org.eclipse.swt.widgets.Shell;
/**
* ZetCode Java SWT tutorial
*
* This program creates a check menu item.
* It will show or hide a statusbar.
*
* @author jan bodnar
* website zetcode.com
* last modified June 2009
*/
public class SWTApp {
private Shell shell;
private Label status;
private MenuItem statItem;
public SWTApp(Display display) {
shell = new Shell(display);
shell.setText("Check menu item");
initUI();
shell.setSize(300, 250);
shell.setLocation(300, 300);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
}
Listener statListener = new Listener() {
public void handleEvent(Event event) {
if (statItem.getSelection()) {
status.setVisible(true);
} else {
status.setVisible(false);
}
}
};
public void initUI() {
Menu menuBar = new Menu(shell, SWT.BAR);
shell.setMenuBar(menuBar);
MenuItem cascadeFileMenu = new MenuItem(menuBar, SWT.CASCADE);
cascadeFileMenu.setText("&File");
Menu fileMenu = new Menu(shell, SWT.DROP_DOWN);
cascadeFileMenu.setMenu(fileMenu);
MenuItem exitItem = new MenuItem(fileMenu, SWT.PUSH);
exitItem.setText("&Exit");
MenuItem cascadeViewMenu = new MenuItem(menuBar, SWT.CASCADE);
cascadeViewMenu.setText("&View");
Menu viewMenu = new Menu(shell, SWT.DROP_DOWN);
cascadeViewMenu.setMenu(viewMenu);
statItem = new MenuItem(viewMenu, SWT.CHECK);
statItem.setSelection(true);
statItem.setText("&View Statusbar");
statItem.addListener(SWT.Selection, statListener);
exitItem.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
shell.getDisplay().dispose();
System.exit(0);
}
});
status = new Label(shell, SWT.BORDER);
status.setText("Ready");
FormLayout layout = new FormLayout();
shell.setLayout(layout);
FormData labelData = new FormData();
labelData.left = new FormAttachment(0);
labelData.right = new FormAttachment(100);
labelData.bottom = new FormAttachment(100);
status.setLayoutData(labelData);
}
public static void main(String[] args) {
Display display = new Display();
new SWTApp(display);
display.dispose();
}
}
statItem = new MenuItem(viewMenu, SWT.CHECK);The
SWT.CHECK
flag will create a check menu item. statItem.setSelection(true);The
setSelection()
method checks/unchecks the check menu item. if (statItem.getSelection()) {Depending on the state of the check menu item, we show or hide the statusbar widget.
status.setVisible(true);
} else {
status.setVisible(false);
}
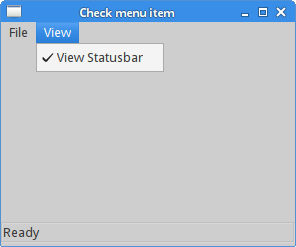
Figure: CheckMenuItem
Popup menu
In the next example, we will create a popup menu. It is also called a context menu. This type of menu is shown, when we right click on an object.package com.zetcode;In our code example, we create a popup menu with two menu items. The first will minimize the window and the second will terminate the application.
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Menu;
import org.eclipse.swt.widgets.MenuItem;
import org.eclipse.swt.widgets.Shell;
/**
* ZetCode Java SWT tutorial
*
* This program creates a popup
* menu
*
* @author jan bodnar
* website zetcode.com
* last modified June 2009
*/
public class SWTApp {
private Shell shell;
public SWTApp(Display display) {
shell = new Shell(display);
shell.setText("Popup menu");
initUI();
shell.setSize(300, 250);
shell.setLocation(300, 300);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
}
public void initUI() {
Menu menu = new Menu(shell, SWT.POP_UP);
MenuItem minItem = new MenuItem(menu, SWT.PUSH);
minItem.setText("Minimize");
minItem.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
shell.setMinimized(true);
}
});
MenuItem exitItem = new MenuItem(menu, SWT.PUSH);
exitItem.setText("Exit");
exitItem.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
shell.getDisplay().dispose();
System.exit(0);
}
});
shell.setMenu(menu);
}
public static void main(String[] args) {
Display display = new Display();
new SWTApp(display);
display.dispose();
}
}
Menu menu = new Menu(shell, SWT.POP_UP);Popup menu is created with the
SWT.POP_UP
flag. MenuItem minItem = new MenuItem(menu, SWT.PUSH);Menu items inside a popup menu are normal push menu items.
minItem.setText("Minimize");
public void widgetSelected(SelectionEvent e) {This code will minimize the window.
shell.setMinimized(true);
}
shell.setMenu(menu);We set a popup menu for the shell.
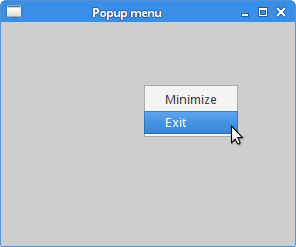
Figure: Popup menu
Simple toolbar
Menus group commands that we can use in application. Toolbars provide a quick access to the most frequently used commands. In the following example, we will create a simple toolbar.package com.zetcode;The example shows a toolbar and three tool items.
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.graphics.Device;
import org.eclipse.swt.graphics.Image;
import org.eclipse.swt.widgets.Display;
import org.eclipse.swt.widgets.Shell;
import org.eclipse.swt.widgets.ToolBar;
import org.eclipse.swt.widgets.ToolItem;
/**
* ZetCode Java SWT tutorial
*
* This program creates a simple toolbar
*
* @author jan bodnar
* website zetcode.com
* last modified June 2009
*/
public class SWTApp {
private Shell shell;
private Image newi;
private Image opei;
private Image quii;
public SWTApp(Display display) {
shell = new Shell(display);
shell.setText("Simple toolbar");
initUI();
shell.setSize(300, 250);
shell.setLocation(300, 300);
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
}
public void initUI() {
Device dev = shell.getDisplay();
try {
newi = new Image(dev, "new.png");
opei = new Image(dev, "open.png");
quii = new Image(dev, "quit.png");
} catch (Exception e) {
System.out.println("Cannot load images");
System.out.println(e.getMessage());
System.exit(1);
}
ToolBar toolBar = new ToolBar(shell, SWT.BORDER);
ToolItem item1 = new ToolItem(toolBar, SWT.PUSH);
item1.setImage(newi);
ToolItem item2 = new ToolItem(toolBar, SWT.PUSH);
item2.setImage(opei);
new ToolItem(toolBar, SWT.SEPARATOR);
ToolItem item3 = new ToolItem(toolBar, SWT.PUSH);
item3.setImage(quii);
toolBar.pack();
item3.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent e) {
shell.getDisplay().dispose();
System.exit(0);
}
});
}
public static void main(String[] args) {
Display display = new Display();
new SWTApp(display);
display.dispose();
}
}
ToolBar toolBar = new ToolBar(shell, SWT.BORDER);Toolbar widget is created.
ToolItem item1 = new ToolItem(toolBar, SWT.PUSH);We create a tool item with an image.
item1.setImage(newi);
new ToolItem(toolBar, SWT.SEPARATOR);Here we create a vertical separator.

Figure: Toolbar
In this chapter of the Java SWT tutorial, we showed, how to work with menus & toolbars.
0 comments:
Post a Comment