PyCairo backends
PyCairo supports various backends. Backends are places where the graphics produced by PyCairo can be displayed. We will use PyCairo to create a PNG image, a PDF file, a SVG file and we will draw on a GTK window.PNG image
In the first example, we will create a PNG image.#!/usr/bin/pythonThis example is a small console application that will create a PNG image.
'''
ZetCode PyCairo tutorial
This program uses PyCairo to
produce a PNG image.
author: Jan Bodnar
website: zetcode.com
last edited: August 2012
'''
import cairo
def main():
ims = cairo.ImageSurface(cairo.FORMAT_ARGB32, 390, 60)
cr = cairo.Context(ims)
cr.set_source_rgb(0, 0, 0)
cr.select_font_face("Sans", cairo.FONT_SLANT_NORMAL,
cairo.FONT_WEIGHT_NORMAL)
cr.set_font_size(40)
cr.move_to(10, 50)
cr.show_text("Disziplin ist Macht.")
ims.write_to_png("image.png")
if __name__ == "__main__":
main()
import cairoWe import the PyCairo module.
ims = cairo.ImageSurface(cairo.FORMAT_ARGB32, 390, 60)We create a surface and a Cairo context from the surface. The surface is a 390x60px image.
cr = cairo.Context(ims)
cr.set_source_rgb(0, 0, 0)We will draw our text in black ink.
cr.select_font_face("Sans", cairo.FONT_SLANT_NORMAL,We choose a font type and set its size.
cairo.FONT_WEIGHT_NORMAL)
cr.set_font_size(40)
cr.move_to(10, 50)We move to a x=10.0, y=50.0 position within the image and draw the text.
cr.show_text("Disziplin ist Macht.")
ims.write_to_png("image.png")This method call creates the PNG image.
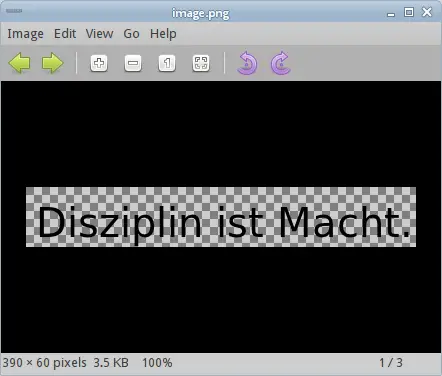
Figure: PNG image in Eye of Gnome
PDF file
In the second example, we create a simple PDF file.#!/usr/bin/pythonWe must open the pdf file in a pdf viewer. Linux users can use KPDF or Evince viewers.
'''
ZetCode PyCairo tutorial
This program uses PyCairo to
produce a PDF image.
author: Jan Bodnar
website: zetcode.com
last edited: August 2012
'''
import cairo
def main():
ps = cairo.PDFSurface("pdffile.pdf", 504, 648)
cr = cairo.Context(ps)
cr.set_source_rgb(0, 0, 0)
cr.select_font_face("Sans", cairo.FONT_SLANT_NORMAL,
cairo.FONT_WEIGHT_NORMAL)
cr.set_font_size(40)
cr.move_to(10, 50)
cr.show_text("Disziplin ist Macht.")
cr.show_page()
if __name__ == "__main__":
main()
ps = cairo.PDFSurface("pdffile.pdf", 504, 648)To render a pdf file, we must create a pdf surface using the
cairo.PDFSurface
object. The size of the pdf file is specified in points, which is a standard in typesetting. cr.show_page()The
show_page()
finishes rendering of the pdf file. 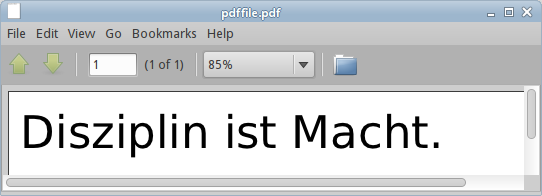
Figure: PDF file in Evince
SVG file
The next example creates a simple SVG (Scalable Vector Graphics) file. A SVG file is a XML based file format.#!/usr/bin/pythonWe can use a web browser like Google Chrome or a vector drawing program like Inkscape to open a SVG file.
'''
ZetCode PyCairo tutorial
This program uses PyCairo to
produce a SVG file.
author: Jan Bodnar
website: zetcode.com
last edited: August 2012
'''
import cairo
def main():
ps = cairo.SVGSurface("svgfile.svg", 390, 60)
cr = cairo.Context(ps)
cr.set_source_rgb(0, 0, 0)
cr.select_font_face("Sans", cairo.FONT_SLANT_NORMAL,
cairo.FONT_WEIGHT_NORMAL)
cr.set_font_size(40)
cr.move_to(10, 50)
cr.show_text("Disziplin ist Macht.")
cr.show_page()
if __name__ == "__main__":
main()
ps = cairo.SVGSurface("svgfile.svg", 390, 60)To create a SVG file in PyCairo, we must create a svg surface using the
cairo.SVGSurface
object. cr.show_page()The
show_page()
method call finisheds rendering of the SVG file. 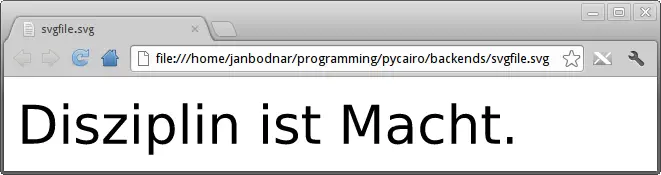
SVG file in Chrome
GTK Window
In the last example, we will draw on a GTK window. This backend will be used throughout the rest of the tutorial.#!/usr/bin/pythonThe example pops up a centered GTK window, on which we draw the "Disziplin ist Macht" text.
'''
ZetCode PyCairo tutorial
This program uses PyCairo to
draw on a window in GTK.
author: Jan Bodnar
website: zetcode.com
last edited: August 2012
'''
from gi.repository import Gtk
import cairo
class Example(Gtk.Window):
def __init__(self):
super(Example, self).__init__()
self.init_ui()
def init_ui(self):
darea = Gtk.DrawingArea()
darea.connect("draw", self.on_draw)
self.add(darea)
self.set_title("GTK window")
self.resize(420, 120)
self.set_position(Gtk.WindowPosition.CENTER)
self.connect("delete-event", Gtk.main_quit)
self.show_all()
def on_draw(self, wid, cr):
cr.set_source_rgb(0, 0, 0)
cr.select_font_face("Sans", cairo.FONT_SLANT_NORMAL,
cairo.FONT_WEIGHT_NORMAL)
cr.set_font_size(40)
cr.move_to(10, 50)
cr.show_text("Disziplin ist Macht.")
def main():
app = Example()
Gtk.main()
if __name__ == "__main__":
main()
from gi.repository import GtkWe import the necessary PyCairo and GTK modules.
import cairo
darea = Gtk.DrawingArea()We will be drawing on a
Gtk.DrawingArea
widget. darea.connect("draw", self.on_draw)When the window is redrawn, a
draw
signal is emitted. We connect that signal to the on_draw()
callback. def on_draw(self, wid, cr):The drawing is done inside the on_draw() method. The third parameter is the cairo context. It is automatically available to us. The Cairo library is built into the GTK system.
...
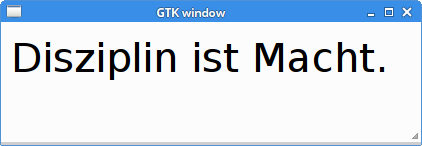
Figure: GTK window
In this chapter we have covered supported PyCairo backends.
0 comments:
Post a Comment