Java developers can choose among several different IDEs. (Integrated Development Environment)
We unzip the file to our preferred directory. Let's say ~/jdeveloper. Now we can lauch the JDeveloper IDE. The startup file is in the ~/jdeveloper/jdev/bin directory.
Type the full pathname of a J2SE installation (or Ctrl-C to quit), the path will be stored in ~/.jdev_jdk
On my system it is /home/vronskij/bin/jdk1.6.0_01. The path will be stored in the hidden .jdev_jdk file name located in the home directory. Then we should see this splashscreen.
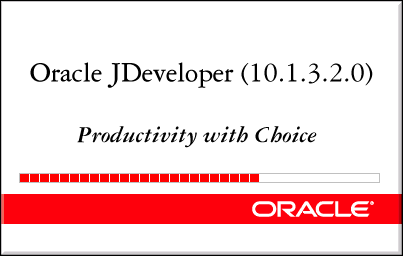
Whoops. A dialog window pops up.
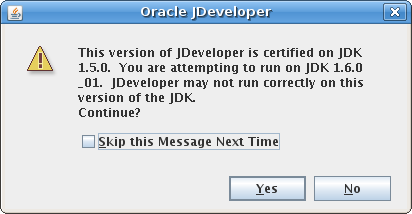
The problem is that I have the newest Java jdk1.6. And the JDeveloper is certified to run on the previous one. I have never encountered any problems regarding this issue. And there is a certain progress from the previous version of JDeveloper. We can grab the dialog box from behind the splashscreen. And click the skip message option.
Now we have been rewarded for our patience and hard work and we have the JDeveloper in full beauty. Ok. One more step. Close the Tip of the day dialog box. And do not forget to unclick the Show tips on startup box. Here we have a screenshot of our beautiful Oracle JDeveloper IDE version 10.1.3.2.0.
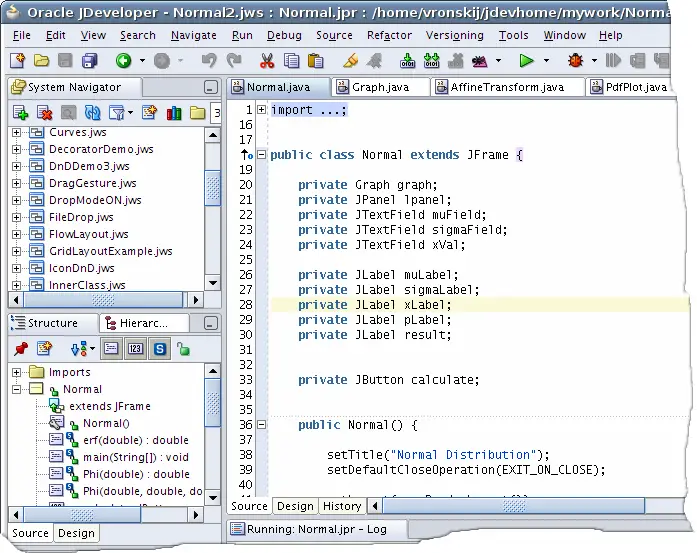
- Eclipse
- Netbeans
- JDeveloper
- JCreator
- IntelliJIDEA
Installation
First we download the JDeveloper IDE from the official download page. We must go through registration process to access the download file. It is a bit annoying. Especially, when you want to uprade your version and you have forgotten your password or login. (or both). I am lost in all those logins and passwords. At home, at work, on the web. The current version is 10.1.3.2. It was realesed in January 2007. I have downloaded the basic java edition. It is perfect for my development of small desktop applications in Java and Swing. There are also three install types. Windows, Mac and universal base install. I work on linux, so I have chosen the base install. It works on all platforms. The other two are probably optimized for those two plaforms. I assume. The dowload file name is jdevjavabase10132.zip.We unzip the file to our preferred directory. Let's say ~/jdeveloper. Now we can lauch the JDeveloper IDE. The startup file is in the ~/jdeveloper/jdev/bin directory.
./jdevWhen we first launch the JDeveloper, it will ask for the path to the Java jdk directory.
Type the full pathname of a J2SE installation (or Ctrl-C to quit), the path will be stored in ~/.jdev_jdk
On my system it is /home/vronskij/bin/jdk1.6.0_01. The path will be stored in the hidden .jdev_jdk file name located in the home directory. Then we should see this splashscreen.
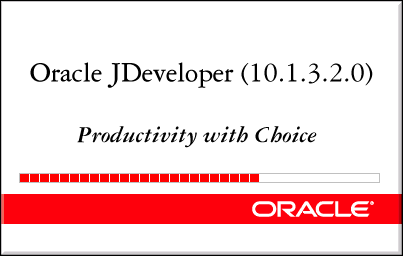
Whoops. A dialog window pops up.
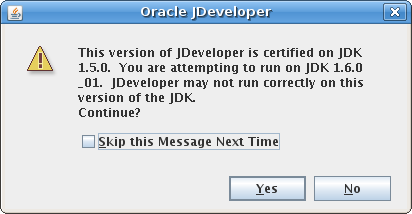
The problem is that I have the newest Java jdk1.6. And the JDeveloper is certified to run on the previous one. I have never encountered any problems regarding this issue. And there is a certain progress from the previous version of JDeveloper. We can grab the dialog box from behind the splashscreen. And click the skip message option.
Now we have been rewarded for our patience and hard work and we have the JDeveloper in full beauty. Ok. One more step. Close the Tip of the day dialog box. And do not forget to unclick the Show tips on startup box. Here we have a screenshot of our beautiful Oracle JDeveloper IDE version 10.1.3.2.0.
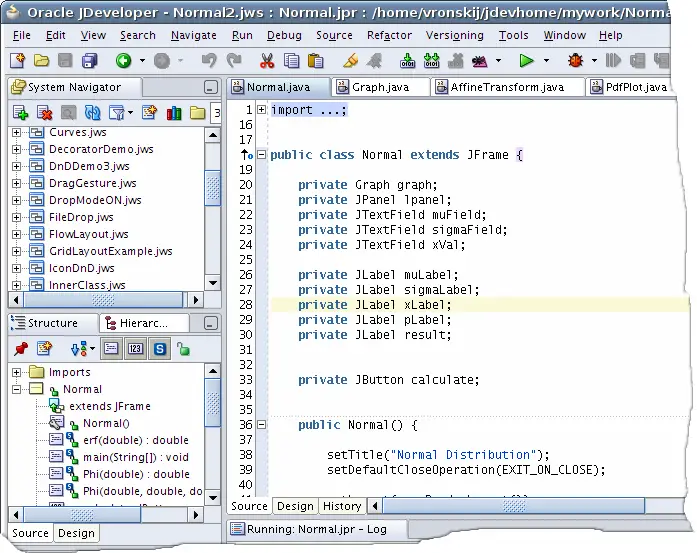
Oracle JDeveloper IDE
Creating a Swing application
I like this JDeveloper slim release, because the creation process is quite simple. (On the other hand, it could be even simpler.) Comparing to other IDE's it is. We do not have to go through all those enterprise apps, beans, libs and the like. We go to File menu, click New. Then we select Application. We give an application a name. In the next dialog window, we provide a name for a project that will host our application. We then go to the System Navigator. Select the project. Right click on the project name, select New Class. We are ready to start coding our Java Swing application.import java.awt.BorderLayout;We have used a 308 x 238px image of Sid. Sid is one of the main characters in the Ice Age movie.
import java.awt.Dimension;
import java.awt.GridLayout;
import java.awt.Image;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.image.CropImageFilter;
import java.awt.image.FilteredImageSource;
import javax.swing.Box;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
public class Puzzle extends JFrame implements ActionListener {
private JPanel centerPanel;
private JButton button;
private JLabel label;
private Image source;
private Image image;
int[][] pos;
public Puzzle() {
pos = new int[][] {
{0, 1, 2},
{3, 4, 5},
{6, 7, 8},
{9, 10, 11}
};
centerPanel = new JPanel();
centerPanel.setLayout(new GridLayout(4, 4, 0, 0));
ImageIcon sid = new ImageIcon(Puzzle.class.getResource("icesid.jpg"));
source = sid.getImage();
add(Box.createRigidArea(new Dimension(0, 5)), BorderLayout.NORTH);
add(centerPanel, BorderLayout.CENTER);
for ( int i = 0; i < 4; i++) {
for ( int j = 0; j < 3; j++) {
if ( j == 2 && i == 3) {
label = new JLabel("");
centerPanel.add(label);
} else {
button = new JButton();
button.addActionListener(this);
centerPanel.add(button);
image = createImage(new FilteredImageSource(source.getSource(),
new CropImageFilter(j*103, i*60, 103, 60)));
button.setIcon(new ImageIcon(image));
}
}
}
setSize(325, 275);
setTitle("Puzzle");
setResizable(false);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
setVisible(true);
}
public static void main(String[] args) {
new Puzzle();
}
public void actionPerformed(ActionEvent e) {
JButton button = (JButton) e.getSource();
Dimension size = button.getSize();
int labelX = label.getX();
int labelY = label.getY();
int buttonX = button.getX();
int buttonY = button.getY();
int buttonPosX = buttonX / size.width;
int buttonPosY = buttonY / size.height;
int buttonIndex = pos[buttonPosY][buttonPosX];
if (labelX == buttonX && (labelY - buttonY) == size.height ) {
int labelIndex = buttonIndex + 3;
centerPanel.remove(buttonIndex);
centerPanel.add(label, buttonIndex);
centerPanel.add(button,labelIndex);
centerPanel.validate();
}
if (labelX == buttonX && (labelY - buttonY) == -size.height ) {
int labelIndex = buttonIndex - 3;
centerPanel.remove(labelIndex);
centerPanel.add(button,labelIndex);
centerPanel.add(label, buttonIndex);
centerPanel.validate();
}
if (labelY == buttonY && (labelX - buttonX) == size.width ) {
int labelIndex = buttonIndex + 1;
centerPanel.remove(buttonIndex);
centerPanel.add(label, buttonIndex);
centerPanel.add(button,labelIndex);
centerPanel.validate();
}
if (labelY == buttonY && (labelX - buttonX) == -size.width ) {
int labelIndex = buttonIndex - 1;
centerPanel.remove(buttonIndex);
centerPanel.add(label, labelIndex);
centerPanel.add(button,labelIndex);
centerPanel.validate();
}
}
}
0 comments:
Post a Comment