Introduction to JRuby Swing
In this part of the JRuby Swing tutorial, we will introduce the Swing toolkit and create our first programs using the JRuby programming language.The purpose of this tutorial is to get you started with the Swing toolkit with the JRuby language. Images used in this tutorial can be downloaded here. I used some icons from the Tango icons pack of the Gnome project.
About
Swing library is an official Java GUI toolkit for the Java programming language. It is used to create Graphical user interfaces with Java. Swing is an advanced GUI toolkit. It has a rich set of components. From basic ones like buttons, labels, scrollbars to advanced components like trees and tables. Swing itself is written in Java. Swing is available for other languages too. For example JRuby, Jython, Groovy or Scala.JRuby is a Java implementation of the Ruby programming language. JRuby can import any Java class.
There are two basic ways to execute the examples in this tutorial. One way is to install a Ruby NetBeans plugin. It contains JRuby as well. When you create a new Ruby project, be sure to select the JRuby platform.
The other way is to download a release from the jruby.orgwebsite.
$ tar -xzvf jruby-bin-1.5.6.tar.gzInstalling JRuby is very easy. We extract the contents of the compressed archive and move the directory to a selected location. On my system, I have moved the directory to the bin directory of my home directory.
$ mv jruby-1.5.6/ ~/bin
$ ~/bin/jdk1.6.0_21/bin/java -jar ~/bin/jruby-1.5.6/lib/jruby.jar simple.rbWe have installed JRuby in a selected directory. In the lib subdirectory, we will find jruby.jar file, which is used to execute JRuby scripts.
$ cat /usr/local/bin/jrubyOptionally, we can create a bash file which will automatically start our JRuby scripts. We can then put the #!/usr/local/bin/jruby path to our scripts.
#!/bin/bash
~/bin/jdk1.6.0_21/bin/java -jar ~/bin/jruby-1.5.6/lib/jruby.jar $1
Simple example
In our first example, we will show a basic window on the screen.#!/usr/local/bin/jrubyWhile this code is very small, the application window can do quite a lot. It can be resized, maximized, minimized. All the complexity that comes with it has been hidden from the application programmer.
# ZetCode JRuby Swing tutorial
#
# This example shows a simple
# window in the center of the screen.
#
# author: Jan Bodnar
# website: www.zetcode.com
# last modified: December 2010
include Java
import javax.swing.JFrame
class Example < JFrame
def initialize
super "Simple"
self.initUI
end
def initUI
self.setSize 300, 200
self.setDefaultCloseOperation JFrame::EXIT_ON_CLOSE
self.setLocationRelativeTo nil
self.setVisible true
end
end
Example.new
include JavaWe include Java API to JRuby.
import javax.swing.JFrameWe import a
JFrame
class. The JFrame
is a top-level window with a titlebar and a border. self.initUIWe delegate the creation of the user interface to the
initUI
method. self.setSize 300, 200We set the size of the window.
self.setDefaultCloseOperation JFrame::EXIT_ON_CLOSEThis method ensures that the window terminates, if we click on the close button of the titlebar. By default nothing happens.
self.setLocationRelativeTo nilWe center the window on the screen.
self.setVisible trueFinally, the window is showed on the screen.
Tooltip
A tooltip is a small rectangular window, which gives a brief information about an object. It is usually a GUI component. It is part of the help system of the application.#!/usr/local/bin/jrubyIn the example, we set the tooltip for the frame and the button.
# ZetCode JRuby Swing tutorial
#
# This code shows a tooltip on
# a window and a button
#
# author: Jan Bodnar
# website: www.zetcode.com
# last modified: December 2010
include Java
import javax.swing.JButton
import javax.swing.JFrame
import javax.swing.JPanel
class Example < JFrame
def initialize
super "Tooltips"
self.initUI
end
def initUI
panel = JPanel.new
self.getContentPane.add panel
panel.setLayout nil
panel.setToolTipText "A Panel container"
button = JButton.new "Button"
button.setBounds 100, 60, 100, 30
button.setToolTipText "A button component"
panel.add button
self.setDefaultCloseOperation JFrame::EXIT_ON_CLOSE
self.setSize 300, 200
self.setLocationRelativeTo nil
self.setVisible true
end
end
Example.new
panel = JPanel.newWe create a
self.getContentPane.add panel
JPanel
component. It is a generic lightweight container. JFrame
has an area, where you put the components called the content pane. We put the panel into this pane. panel.setLayout nilBy default, the
JPanel
has a FlowLayout
manager. The layout manager is used to place widgets onto the containers. If we call setLayout nil
we can position our components absolutely. For this, we use the setBounds
method. panel.setToolTipText "A Panel container"To enable a tooltip, we call the
setTooltipText
method. 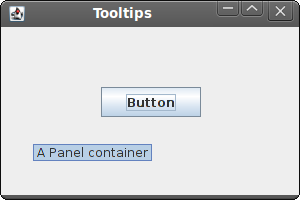
Figure: Tooltip
Quit button
In the last example of this section, we will create a quit button. When we press this button, the application terminates.#!/usr/local/bin/jrubyWe position a
# ZetCode JRuby Swing tutorial
#
# This program creates a quit
# button. When we press the button,
# the application terminates.
#
# author: Jan Bodnar
# website: www.zetcode.com
# last modified: December 2010
include Java
import javax.swing.JButton
import javax.swing.JFrame
import javax.swing.JPanel
import java.lang.System
class Example < JFrame
def initialize
super "Quit button"
self.initUI
end
def initUI
panel = JPanel.new
self.getContentPane.add panel
panel.setLayout nil
qbutton = JButton.new "Quit"
qbutton.setBounds 50, 60, 80, 30
qbutton.add_action_listener do |e|
System.exit 0
end
panel.add qbutton
self.setDefaultCloseOperation JFrame::EXIT_ON_CLOSE
self.setSize 300, 200
self.setLocationRelativeTo nil
self.setVisible true
end
end
Example.new
JButton
on the window. We will add an action listener to this button. qbutton = JButton.new "Quit"Here we create a button. We position it by calling the
qbutton.setBounds 50, 60, 80, 30
setBounds
method. qbutton.add_action_listener do |e|We add an action listener to the button. The listener terminates the application.
System.exit 0
end
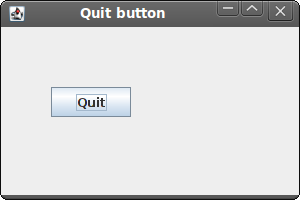
Figure: Quit button
This section was an introduction to the Swing toolkit with the JRuby language.
0 comments:
Post a Comment