Nibbles
In this part of the JRuby Swing programming tutorial, we will create a Nibbles game clone.Nibbles is an older classic video game. It was first created in late 70s. Later it was brought to PCs. In this game the player controls a snake. The objective is to eat as many apples as possible. Each time the snake eats an apple, its body grows. The snake must avoid the walls and its own body.
Development
The size of each of the joints of a snake is 10px. The snake is controlled with the cursor keys. Initially, the snake has three joints. The game starts immediately. When the game is finished, we display "Game Over" message in the center of the window.#!/usr/local/bin/jrubyFirst we will define some constants used in our game.
# ZetCode JRuby Swing tutorial
#
# In this program, we create
# a Nibbles game clone.
#
# author: Jan Bodnar
# website: www.zetcode.com
# last modified: December 2010
include Java
import java.awt.Color
import java.awt.Font
import java.awt.Dimension
import java.awt.Toolkit
import java.awt.event.ActionListener
import java.awt.event.KeyEvent
import java.awt.event.KeyListener
import javax.swing.JFrame
import javax.swing.ImageIcon
import javax.swing.JPanel
import javax.swing.Timer
NWIDTH = 300
NHEIGHT = 300
DOT_SIZE = 10
ALL_DOTS = NWIDTH * NHEIGHT / (DOT_SIZE * DOT_SIZE)
RAND_POS = 25
DELAY = 140
$x = [0] * ALL_DOTS
$y = [0] * ALL_DOTS
class Board < JPanel
include KeyListener, ActionListener
def initialize
super
self.setFocusable true
self.initGame
end
def initGame
@left = false
@right = true
@up = false
@down = false
@inGame = true
@dots = 3
begin
iid = ImageIcon.new "dot.png"
@ball = iid.getImage
iia = ImageIcon.new "apple.png"
@apple = iia.getImage
iih = ImageIcon.new "head.png"
@head = iih.getImage
rescue
puts "cannot load images"
end
for i in 0..@dots
$x[i] = 50 - i * 10
$y[i] = 50
end
self.locateApple
self.setBackground Color.black
self.addKeyListener self
@timer = Timer.new DELAY, self
@timer.start
end
def paint g
super g
if @inGame
self.drawObjects g
Toolkit.getDefaultToolkit.sync
g.dispose
else
self.gameOver g
end
end
def drawObjects g
g.drawImage @apple, @apple_x, @apple_y, self
for z in 0..@dots
if z == 0
g.drawImage @head, $x[z], $y[z], self
else
g.drawImage @ball, $x[z], $y[z], self
end
end
end
def gameOver g
msg = "Game Over"
small = Font.new "Helvetica", Font::BOLD, 14
metr = self.getFontMetrics small
g.setColor Color.white
g.setFont small
g.drawString msg, (NWIDTH - metr.stringWidth(msg)) / 2,
NHEIGHT / 2
@timer.stop
end
def checkApple
if $x[0] == @apple_x and $y[0] == @apple_y
@dots = @dots + 1
self.locateApple
end
end
def move
z = @dots
while z > 0
$x[z] = $x[(z - 1)]
$y[z] = $y[(z - 1)]
z = z - 1
end
if @left
$x[0] -= DOT_SIZE
end
if @right
$x[0] += DOT_SIZE
end
if @up
$y[0] -= DOT_SIZE
end
if @down
$y[0] += DOT_SIZE
end
end
def checkCollision
z = @dots
while z > 0
if z > 4 and $x[0] == $x[z] and $y[0] == $y[z]
@inGame = false
end
z = z - 1
end
if $y[0] > NHEIGHT - DOT_SIZE
@inGame = false
end
if $y[0] < 0
@inGame = false
end
if $x[0] > NWIDTH - DOT_SIZE
@inGame = false
end
if $x[0] < 0
@inGame = false
end
end
def locateApple
r = rand RAND_POS
@apple_x = r * DOT_SIZE
r = rand RAND_POS
@apple_y = r * DOT_SIZE
end
def actionPerformed e
if @inGame
self.checkApple
self.checkCollision
self.move
end
self.repaint
end
def keyReleased e
end
def keyPressed e
key = e.getKeyCode
if key == KeyEvent::VK_LEFT and not @right
@left = true
@up = false
@down = false
end
if key == KeyEvent::VK_RIGHT and not @left
@right = true
@up = false
@down = false
end
if key == KeyEvent::VK_UP and not @down
@up = true
@right = false
@left = false
end
if key == KeyEvent::VK_DOWN and not @up
@down = true
@right = false
@left = false
end
end
end
class Example < JFrame
def initialize
super "Nibbles"
self.initUI
end
def initUI
board = Board.new
board.setPreferredSize Dimension.new NWIDTH, NHEIGHT
self.add board
self.pack
self.setResizable false
self.setDefaultCloseOperation JFrame::EXIT_ON_CLOSE
self.setLocationRelativeTo nil
self.setVisible true
end
end
Example.new
The
WIDTH
and HEIGHT
constants determine the size of the Board. The DOT_SIZE
is the size of the apple and the dot of the snake. The ALL_DOTS
constant defines the maximum number of possible dots on the Board. The RAND_POS
constant is used to calculate a random position of an apple. The DELAY
constant determines the speed of the game. $x = [0] * ALL_DOTSThese two arrays store x, y coordinates of all possible joints of a snake.
$y = [0] * ALL_DOTS
The
initGame
method initializes variables, loads images and starts a timeout function. def paint gInside the
super g
if @inGame
self.drawObjects g
Toolkit.getDefaultToolkit.sync
g.dispose
else
self.gameOver g
end
end
paint
method, we check the @inGame variable. If it is true, we draw our objects. The apple and the snake joints. Otherwise we display "Game over" text. The Toolkit.getDefaultToolkit.sync
method ensures that the display is up-to-date. It is useful for animation. def drawObjects gThe
g.drawImage @apple, @apple_x, @apple_y, self
for z in 0..@dots
if z == 0
g.drawImage @head, $x[z], $y[z], self
else
g.drawImage @ball, $x[z], $y[z], self
end
end
end
drawObjects
method draws the apple and the joints of the snake. The first joint of a snake is its head, which is represented by a red circle. def gameOver gIn the gameOver method, we display "Game Over" message in the center of the window. We also stop the timer.
msg = "Game Over"
small = Font.new "Helvetica", Font::BOLD, 14
metr = self.getFontMetrics small
g.setColor Color.white
g.setFont small
g.drawString msg, (NWIDTH - metr.stringWidth(msg)) / 2,
NHEIGHT / 2
@timer.stop
end
def checkAppleThe
if $x[0] == @apple_x and $y[0] == @apple_y
@dots = @dots + 1
self.locateApple
end
end
checkApple
method checks, if the snake has hit the apple object. If so, we add another snake joint and call the locateApple
method, which randomly places a new apple object. In the
move
method we have the key algorithm of the game. To understand it, look at how the snake is moving. You control the head of the snake. You can change its direction with the cursor keys. The rest of the joints move one position up the chain. The second joint moves where the first was, the third joint where the second was etc. while z > 0This code moves the joints up the chain.
$x[z] = $x[(z - 1)]
$y[z] = $y[(z - 1)]
z = z - 1
end
if @leftMove the head to the left.
$x[0] -= DOT_SIZE
end
In the
checkCollision
method, we determine if the snake has hit itself or one of the walls. while z > 0Finish the game, if the snake hits one of its joints with the head.
if z > 4 and $x[0] == $x[z] and $y[0] == $y[z]
@inGame = false
end
z = z - 1
end
if $y[0] > NHEIGHT - DOT_SIZEFinish the game, if the snake hits the bottom of the Board.
@inGame = false
end
The
locateApple
method locates an apple randomly on the board. r = rand RAND_POSWe get a random number from 0 to RAND_POS - 1.
@apple_x = r * DOT_SIZEThese lines set the x, y coordinates of the apple object.
...
@apple_y = r * DOT_SIZE
def actionPerformed eEvery DELAY ms, the
if @inGame
self.checkApple
self.checkCollision
self.move
end
self.repaint
end
actionPerformed
method is called. If we are in the game, we call three methods, that build the logic of the game. In the
keyPressed
method of the Board class, we determine the keys that were pressed. if key == KeyEvent::VK_LEFT and not @rightIf we hit the left cursor key, we set
@left = true
@up = false
@down = false
end
@left
variable to true. This variable is used in the move
method to change coordinates of the snake object. Notice also, that when the snake is heading to the right, we cannot turn immediately to the left. class Example < JFrameIn this class, we set up the Nibbles game.
def initialize
super "Nibbles"
self.initUI
end
def initUI
board = Board.new
board.setPreferredSize Dimension.new NWIDTH, NHEIGHT
self.add board
self.pack
self.setResizable false
self.setDefaultCloseOperation JFrame::EXIT_ON_CLOSE
self.setLocationRelativeTo nil
self.setVisible true
end
end
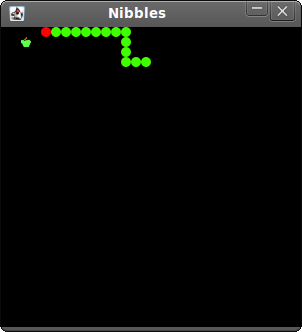
Figure: Nibbles
This was the Nibbles computer game programmed with the Swing library and the JRuby programming language.
0 comments:
Post a Comment